How To Blur Image In Javascript
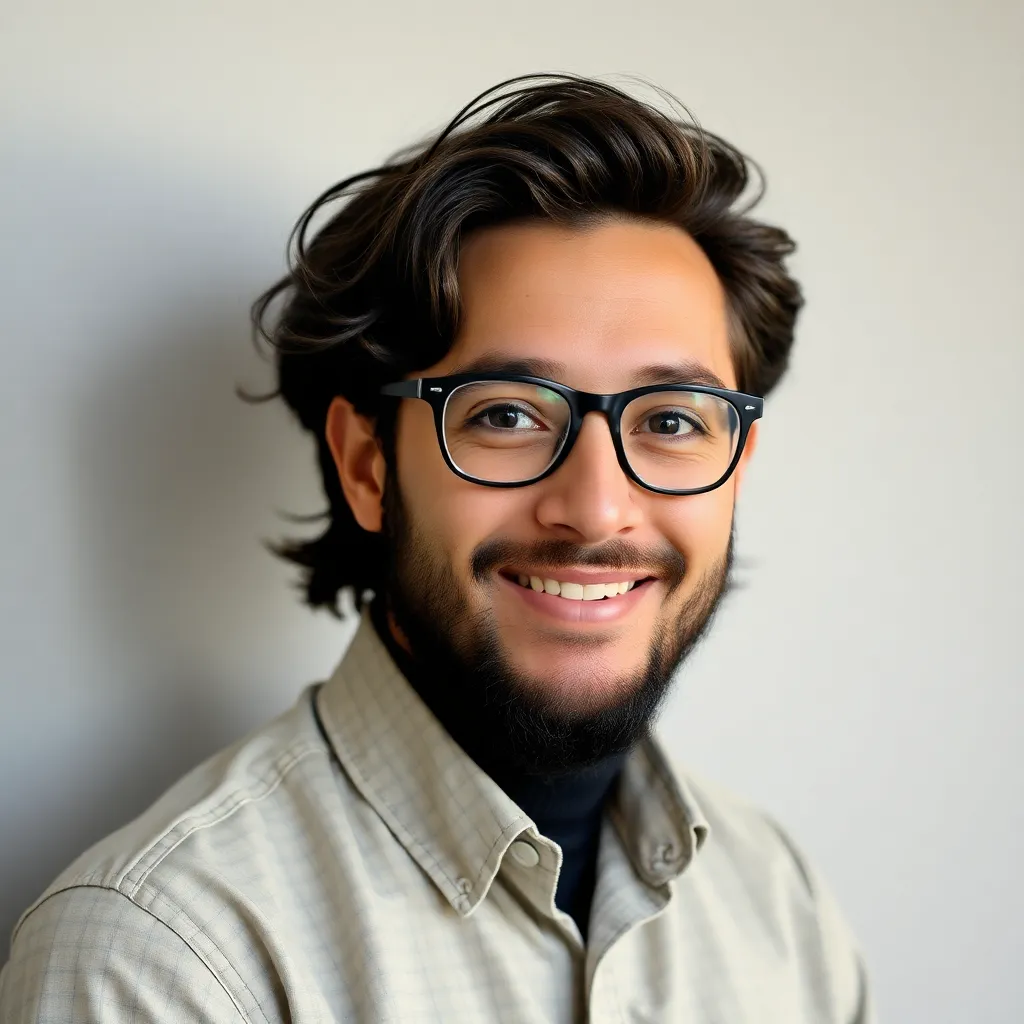
Ronan Farrow
Feb 27, 2025 · 3 min read

Table of Contents
How to Blur an Image in JavaScript: A Comprehensive Guide
Blurring images is a common image processing task with many applications, from creating visually appealing effects to protecting sensitive information. JavaScript, with the help of the HTML5 Canvas API, offers a straightforward way to achieve this. This guide will walk you through several methods for blurring images in JavaScript, from simple box blur to more sophisticated Gaussian blur techniques. We'll cover the code, explain the concepts, and help you choose the best approach for your needs.
Understanding Image Blurring
Before diving into the code, let's briefly understand the concept of image blurring. Essentially, blurring an image involves averaging the colors of neighboring pixels. The greater the area of averaging, the more blurred the image becomes. Different blurring algorithms vary in how they perform this averaging, leading to different blur effects.
Method 1: Basic Box Blur using Canvas
This method is the simplest to implement. It averages the color values of pixels within a defined square area (the "box"). It's computationally inexpensive but produces a less refined blur compared to more advanced techniques.
Step-by-step implementation:
-
Get the Image: Load your image using an
<img>
tag. You'll need its ID to access it in your JavaScript code. -
Create a Canvas: Create an HTML5 canvas element of the same size as your image. This canvas will be where the blurred image is drawn.
-
Draw the Image onto the Canvas: Use the
drawImage()
method to draw the original image onto the canvas. -
Get Image Data: Use
getImageData()
to retrieve the pixel data from the canvas as aImageData
object. This object contains an array representing the pixel data (RGBA values). -
Apply the Box Blur: This is where the core blurring logic resides. For each pixel, average the color values of its neighboring pixels within the box radius. The larger the radius, the more pronounced the blur.
-
Set Image Data: Update the
ImageData
object with the new blurred pixel data. -
Put Image Data back onto Canvas: Use
putImageData()
to place the modifiedImageData
back onto the canvas, displaying the blurred image.
Code Example (Box Blur):
function boxBlur(imgId, radius) {
const img = document.getElementById(imgId);
const canvas = document.createElement('canvas');
const ctx = canvas.getContext('2d');
canvas.width = img.width;
canvas.height = img.height;
ctx.drawImage(img, 0, 0);
const imgData = ctx.getImageData(0, 0, canvas.width, canvas.height);
const data = imgData.data;
//Box blur logic (simplified for brevity. A complete implementation would require handling edge cases)
for (let i = 0; i < data.length; i += 4) {
// ... (Box blur averaging calculation here) ...
}
ctx.putImageData(imgData, 0, 0);
document.body.appendChild(canvas); // Append the canvas to display the blurred image
}
// Example usage: boxBlur('myImage', 5); // 5 is the blur radius
Note: This code snippet only provides a skeletal structure. The complete box blur calculation (step 5) involves iterating through pixels and averaging their RGB values within the specified radius. This calculation can be computationally intensive for large images and high radii.
Method 2: Gaussian Blur (More Advanced)
Gaussian blur provides a smoother, more natural-looking blur compared to the box blur. It uses a Gaussian function to weight the contribution of neighboring pixels, giving more importance to pixels closer to the center. Implementing a Gaussian blur directly in JavaScript can be complex, often requiring multiple passes or convolutions.
Considerations for Gaussian Blur:
- Complexity: Gaussian blur is significantly more complex than box blur.
- Performance: It's more computationally expensive, especially for large images and large kernel sizes (radius).
- Libraries: Consider using a JavaScript image processing library (like OpenCV.js or a similar library) to handle the computationally intensive Gaussian blur algorithm efficiently. These libraries often provide optimized implementations.
Choosing the Right Method
The best method depends on your needs:
- Simple Blur, Performance is Crucial: Use the box blur.
- High-Quality Blur, Performance is Less Critical: Use a Gaussian blur, potentially leveraging a JavaScript image processing library.
This comprehensive guide provides a foundational understanding of image blurring in JavaScript. Remember to handle edge cases and optimize your code for performance, especially when dealing with larger images. Experiment with different blur radii and methods to achieve the desired effect for your projects.
Featured Posts
Also read the following articles
Article Title | Date |
---|---|
How To Kiss Girl First Time | Feb 27, 2025 |
How To Clear Cache In Chrome Video | Feb 27, 2025 |
How To Activate Windows 7 Reddit | Feb 27, 2025 |
How To Make Pancake Muffins | Feb 27, 2025 |
How To Cancel Spotify Premium After Free Trial | Feb 27, 2025 |
Latest Posts
Thank you for visiting our website which covers about How To Blur Image In Javascript . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.